How to save file with file name from user using Python?
Last Updated :
13 Jan, 2021
Prerequisites:
Saving a file with the user’s custom name can be achieved using python file handling concepts. Python provides inbuilt functions for working with files. The file can be saved with the user preferred name by creating a new file, renaming the existing file, making a copy of a file(Save As). Let’s discuss these in detail.
Creating a new file
Method 1: Using open() function
We can create a new file using the open() function with one of the access modes listed below.
Syntax:
open( filepath , mode )
Access modes:
- Write Only (‘w’): Creates a new file for writing, if the file doesn’t exist otherwise truncates and over-write existing file.
- Write and Read (‘w+’): Creates a new file for reading & writing, if the file doesn’t exist otherwise truncates and over-write existing file.
- Append Only (‘a’): Creates a new file for writing, if the file doesn’t exist otherwise data being written will be inserted at the end of the file.
- Append and Read (‘a+’): Creates a new file for reading & writing, if the file doesn’t exist otherwise data being written will be inserted at the end of the file.
Approach
- Get file name from the user
- Open a file with mentioned access mode
- Create this file with the entered name
Example:
Python3
directory = "D:\gfg\\"
filepath = directory + input ( "Enter filename: " )
with open (filepath, 'w+' ) as fp:
pass
|
Output:
Enter filename: newgfgfile.txt
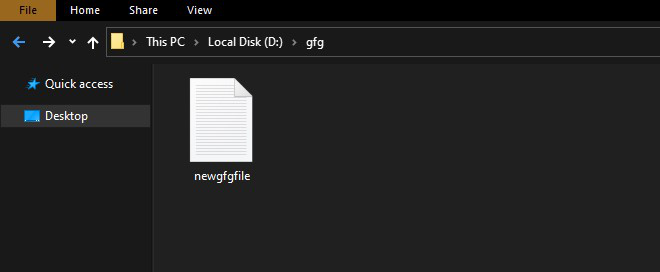
Method 2: Using pathlib library
pathlib offers a set of classes to handle filesystem paths. We can use touch() method to create the file at a given path it updates the file modification time with the current time and marks exist_ok as True, otherwise, FileExistsError is raised.
Syntax:
Path.touch(mode=0o666, exist_ok=True)
Approach
- Import module
- Get file name from the user
- Create a file with the entered name
Example:
Python3
import pathlib
directory = "D:\gfg\\"
filepath = directory + input ( "Enter filename:" )
pathlib.Path(filepath).touch()
|
Output:
Enter filename:gfgfile2.txt
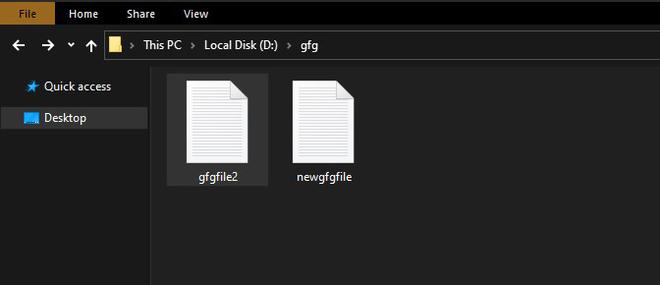
Renaming a file
Method 1: Using the os module
Python’s OS module includes functions to communicate with the operating system. Here, we can use rename() method to save a file with the name specified by the user.
Syntax:
rename(src, dest, *, src_dir_fd=None, dst_dir_fd=None)
Approach:
- Import module
- Get source file name
- Get destination file name
- Rename the source file to destination file or directory
- If destination file already exists, the operation will fail with an OSError.
Example:
Python3
import os
src = input ( "Enter src filename:" )
dest = input ( "Enter dest filename:" )
os.rename(src, dest)
|
Output:
Enter src filename:D:\gfg\newgfgfile.txt
Enter dest filename:D:\gfg\renamedfile1.txt
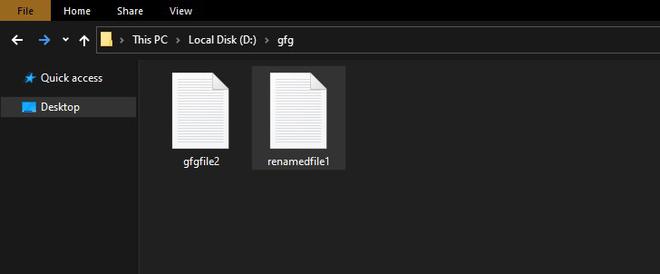
Method 2: Using pathlib library
pathlib also provides rename() function to change the name of a file which more or less serves the same purpose as given above.
syntax:
Path(filepath).rename(target)
Approach:
- Import module
- Get source file name
- Get destination file name
- Rename source file or directory to the destination specified
- Return a new instance of the Path to the destination. (On Unix, if the target exists and the user has permission, it will be replaced.)
Example:
Python3
import pathlib
src = input ( "Enter src filename:" )
target = input ( "Enter target filename:" )
pathlib.Path(src).rename(target)
|
Output:
Enter src filename:D:\gfg\gfgfile2.txt
Enter target filename:D:\gfg\renamedfile2.txt
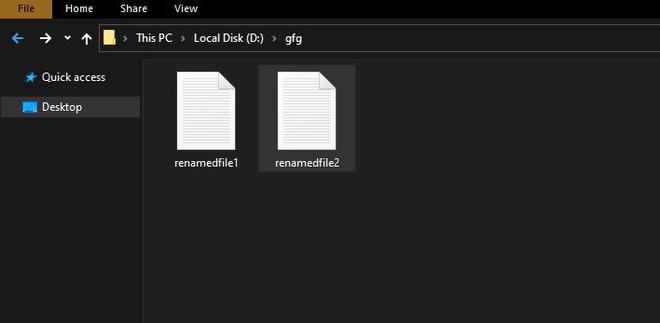
Copying or duplicating a file
Method 1: Using the os module
We can use popen() method to make a copy of the source file to the target file with the name specified by the user.
Syntax:
popen( command, mode , buffersize )
os.popen() get command to be performed as the first argument, access mode as the second argument which can be read (‘r’) or write (‘w’) and finally buffer size. The default mode is read and 0 for no buffering, positive integers for buffer size.
Approach:
- Import module
- Get source file name
- Get destination file name
- Copy source to destination
Example:
Python
import os
src = input ( "Enter src filename:" )
destination = input ( "Enter target filename:" )
os.popen(f "copy {src} {destination}" )
|
Output:
Enter src filename:D:\gfg\renamedfile1.txt
Enter target filename:D:\gfg\copied-renamedfile1.txt
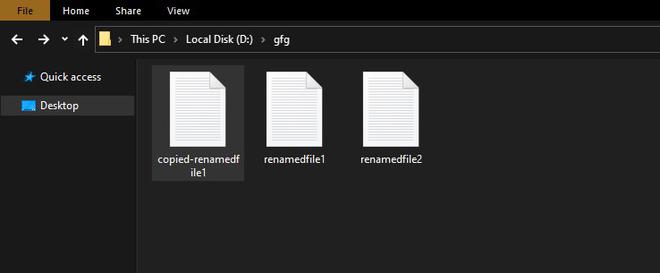
Method 2: Using the shutil module
The shutil module offers several high-level operations on files and collections of files. Its copyfile() method is used to rename the file with the user preferred name.
Syntax:
shutil.copyfile(src_file, dest_file, *, follow_symlinks=True)
Approach:
- Import module
- Get source file name
- Get destination file name
- Copy source file to a new destination file. If both file names specify the same file, SameFileError is raised and if destination file already exists, it will be replaced.
Example:
Python3
import shutil
src = input ( "Enter src filename:" )
dest = input ( "Enter target filename:" )
shutil.copyfile(src, dest)
|
Output:
Enter src filename:D:\gfg\renamedfile2.txt
Enter target filename:D:\gfg\copied-renamedfile2.txt
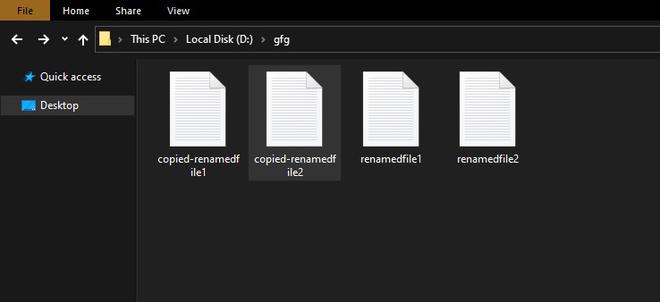
Level up your coding with DSA Python in 90 days! Master key algorithms, solve complex problems, and prepare for top tech interviews. Join the Three 90 Challenge—complete 90% of the course in 90 days and earn a 90% refund. Start your Python DSA journey today!
Similar Reads
NumPy save() Method | Save Array to a File
The NumPy save() method is used to store the input array in a binary file with the 'npy extension' (.npy). Example: Python3 import numpy as np a = np.arange(5) np.save('array_file', a) SyntaxSyntax: numpy.save(file, arr, allow_pickle=True, fix_imports=True) Parameters: file: File or filename to which the data is saved. If the file is a string or P
2 min read
Python PIL save file with datetime as name
In this article, we are going to see how to save image files with datetime as a name using PIL Python. Modules required: PIL: This library provides extensive file format support, an efficient internal representation, and fairly powerful image processing capabilities. pip install Pillow datetime: This module helps us to work with dates and times in
2 min read
Save user input to a Excel File in Python
In this article, we will learn how to store user input in an excel sheet using Python, What is Excel? Excel is a spreadsheet in a computer application that is designed to add, display, analyze, organize, and manipulate data arranged in rows and columns. It is the most popular application for accounting, analytics, data presentation, etc. Methods us
4 min read
Save multiple matplotlib figures in single PDF file using Python
In this article, we will discuss how to save multiple matplotlib figures in a single PDF file using Python. We can use the PdfPages class's savefig() method to save multiple plots in a single pdf. Matplotlib plots can simply be saved as PDF files with the .pdf extension. This saves Matplotlib-generated figures in a single PDF file named Save multip
3 min read
Save Image To File in Python using Tkinter
Saving an uploaded image to a local directory using Tkinter combines the graphical user interface capabilities of Tkinter with the functionality of handling and storing images in Python. In this article, we will explore the steps involved in achieving this task, leveraging Tkinter's GUI features to enhance the user experience in image management ap
4 min read
Scrape and Save Table Data in CSV file using Selenium in Python
Selenium WebDriver is an open-source API that allows you to interact with a browser in the same way a real user would and its scripts are written in various languages i.e. Python, Java, C#, etc. Here we will be working with python to scrape data from tables on the web and store it as a CSV file. As Google Chrome is the most popular browser, to make
3 min read
How to Save a Plot to a File Using Matplotlib?
Matplotlib is a widely used Python library to plot graphs, plots, charts, etc. show() method is used to display graphs as output, but don’t save it in any file. In this article, we will see how to save a Matplotlib plot as an image file. Save a plot in MatplotlibBelow are the ways by which we can save a plot to a file using Matplotlib in Python: Us
3 min read
How To Save The Network In XML File Using PyBrain
In this article, we are going to see how to save the network in an XML file using PyBrain in Python. A network consists of several modules. These modules are generally connected with connections. PyBrain provides programmers with the support of neural networks. A network can be interpreted as an acyclic directed graph where each module serves the p
2 min read
GUI application to search a country name from a given state or city name using Python
In these articles, we are going to write python scripts to search a country from a given state or city name and bind it with the GUI application. We will be using the GeoPy module. GeoPy modules make it easier to locate the coordinates of addresses, cities, countries, landmarks, and Zipcode. Before starting we need to install the GeoPy module, so l
2 min read
How to save a Python Dictionary to a CSV File?
Prerequisites: Working with csv files in Python CSV (comma-separated values) files are one of the easiest ways to transfer data in form of string especially to any spreadsheet program like Microsoft Excel or Google spreadsheet. In this article, we will see how to save a PYthon dictionary to a CSV file. Follow the below steps for the same. Import cs
2 min read