C – Pointer to Pointer (Double Pointer)
Last Updated :
10 Jan, 2025
In C, double pointers are those pointers which stores the address of another pointer. The first pointer is used to store the address of the variable, and the second pointer is used to store the address of the first pointer. That is why they are also known as a pointer to pointer.
Let’s take a look at an example:
C
#include <stdio.h>
int main() {
// A variable
int var = 10;
// Pointer to int
int *ptr1 = &var;
// Pointer to pointer (double pointer)
int **ptr2 = &ptr1;
printf("var: %d\n", var);
printf("*ptr1: %d\n", *ptr1);
printf("**ptr2: %d", **ptr2);
return 0;
}
Outputvar: 10
*ptr1: 10
**ptr2: 10
Explanation: In this code, ptr1 is a pointer that stores the address of the integer variable var. ptr2 is a double pointer that stores the address of the pointer ptr1. **ptr2 dereferences ptr2 to get the value of ptr1 (which is the address of var) and then dereferences that address to get the value of var itself.
Syntax of Double Pointer
The syntax to use double pointer can be divided into three parts:
Declaration
A double pointer can be declared similar to a single pointer. The difference is we have to place an additional ‘*’ before the name of the pointer.
type **name;
Above is the declaration of the double pointer with some name to the given type.
Initialization
The double pointer stores the address of another pointer to the same type.
name = &single_ptr; // After declaration
type **name = &single_ptr; // With declaration
Deferencing
To access the value pointed by double pointer, we have to use the deference operator * two times.
*name; // Gives you the address of the single pointer
**name; // Gives you the value of the variable it points to
The below image illustrates these for the first example:
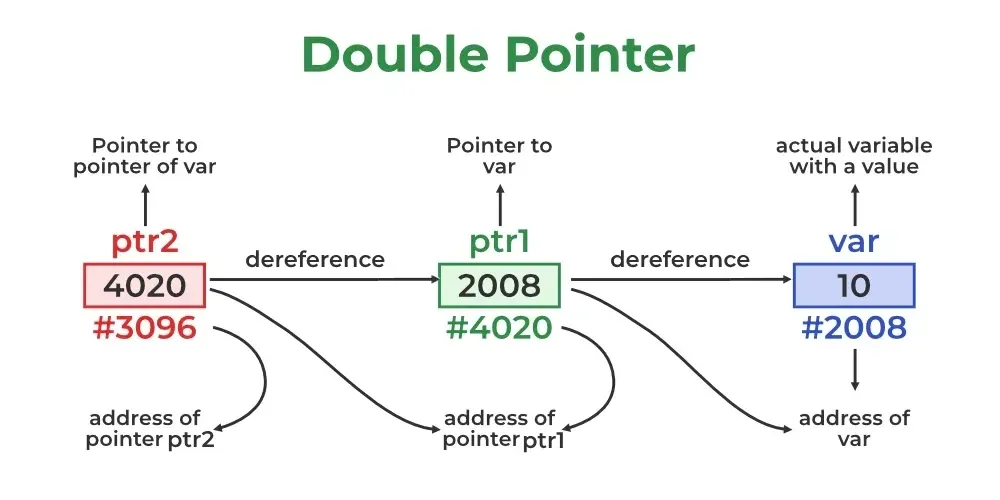
In C, a double pointer behaves similarly to a normal pointer. So, the size of the double-pointer variable is always equal to the normal pointers i.e. only depending on the operating system and CPU architecture.
- 8 bytes for a 64-bit System
- 4 bytes for a 32-bit System
Examples of Double Pointer
The below examples demonstrate the use of double pointers for different applications in C:
Find the Size of Double Pointer
C
#include <stdio.h>
int main() {
// Defining single and double pointers
int a = 5;
int* ptr = &a;
int** d_ptr = &ptr;
// Size of double pointer
printf("%d bytes", sizeof(d_ptr));
return 0;
}
Note: The output of the above code also depends on the type of machine which is being used.
Create a Dynamic 2D Array
C
#include <stdio.h>
#include <stdlib.h>
int main() {
int m = 2, n = 3;
// Create a double pointer
int** arr;
// Allocate memory for rows
arr = (int**)malloc(m * sizeof(int*));
// Allocate memory for each row
for (int i = 0; i < m; i++)
arr[i] = (int*)malloc(n * sizeof(int));
// Initialize with some values
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
arr[i][j] = i * n + j + 1;
}
}
// Print the array
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
printf("%d ", arr[i][j]);
}
printf("\n");
}
// Free allocated memory
for (int i = 0; i < m; i++) {
free(arr[i]);
}
free(arr);
return 0;
}
Explanation: A 2D array is an array where each element is essentially a 1D array. This can be implemented using double pointers. The double pointer points to the first element of the 2D array, and each pointer it references points to a dynamically allocated 1D array using malloc().
Pass Array of Strings to Function
C
#include <stdio.h>
// Function that takes array of strings as argument
void print(char** arr, int n) {
for (int i = 0; i < n; i++)
printf("%s\n", *(arr + i));
}
int main() {
char* arr[10] = {"Geek", "Geeks",
"Geekfor"};
print(arr, 3);
return 0;
}
Explanation: Array of strings are generally stored as array of pointer to strings. This can be passed using double pointers to function.
Application of Double Pointers in C
Following are the main uses of pointer to pointers in C:
- They are used in the dynamic memory allocation of multidimensional arrays.
- They can be used to store multilevel data such as the text document paragraph, sentences, and word semantics.
- They are used in data structures to directly manipulate the address of the nodes without copying.
- They can be used as function arguments to manipulate the address stored in the local pointer.
Multilevel Pointers in C
Double Pointers are not the only multilevel pointers supported by the C language. What if we want to change the value of a double pointer? In this case, we can use a triple pointer, which will be a pointer to a pointer to a pointer i.e, int ***t_ptr.
Examples
int*** ptr3; // Triple pointer
int**** ptr4; // Quadruple Pointer
Similarly, to change the value of a triple pointer we can use a pointer to a pointer to a pointer to a pointer (Four level Pointer). In other words, we can say that to change the value of a ” level – x ” variable we can use a ” level – x+1 ” pointer. And this concept can be extended further.
Note: We can use any level pointer in C. There is no restriction about it but it makes the program very complex and vulnerable to errors.
Similar Reads
C Pointers
A pointer is a variable that stores the memory address of another variable. Instead of holding a direct value, it holds the address where the value is stored in memory. There are 2 important operators that we will use in pointers concepts i.e. Dereferencing operator(*) used to declare pointer variab
10 min read
Pointer Arithmetics in C with Examples
Pointer Arithmetic is the set of valid arithmetic operations that can be performed on pointers. The pointer variables store the memory address of another variable. It doesn't store any value. Hence, there are only a few operations that are allowed to perform on Pointers in C language. The C pointer
10 min read
Applications of Pointers in C
Pointers in C are variables that are used to store the memory address of another variable. Pointers allow us to efficiently manage the memory and hence optimize our program. In this article, we will discuss some of the major applications of pointers in C. Prerequisite: Pointers in C. C Pointers Appl
4 min read
Passing Pointers to Functions in C
Prerequisites: Pointers in CFunctions in C Passing the pointers to the function means the memory location of the variables is passed to the parameters in the function, and then the operations are performed. The function definition accepts these addresses using pointers, addresses are stored using po
2 min read
C - Pointer to Pointer (Double Pointer)
In C, double pointers are those pointers which stores the address of another pointer. The first pointer is used to store the address of the variable, and the second pointer is used to store the address of the first pointer. That is why they are also known as a pointer to pointer. Let's take a look a
5 min read
Chain of Pointers in C with Examples
Prerequisite: Pointers in C, Double Pointer (Pointer to Pointer) in CA pointer is used to point to a memory location of a variable. A pointer stores the address of a variable.Similarly, a chain of pointers is when there are multiple levels of pointers. Simplifying, a pointer points to address of a v
5 min read
Function Pointer in C
In C, a function pointer is a type of pointer that stores the address of a function, allowing functions to be passed as arguments and invoked dynamically. It is useful in techniques such as callback functions, event-driven programs, and polymorphism (a concept where a function or operator behaves di
6 min read
How to Declare a Pointer to a Function?
A pointer to a function is similar to a pointer to a variable. However, instead of pointing to a variable, it points to the address of a function. This allows the function to be called indirectly, which is useful in situations like callback functions or event-driven programming. In this article, we
2 min read
Pointer to an Array | Array Pointer
A pointer to an array is a pointer that points to the whole array instead of the first element of the array. It considers the whole array as a single unit instead of it being a collection of given elements. Consider the following example: [GFGTABS] C #include<stdio.h> int main() { int arr[5] =
5 min read
Difference between constant pointer, pointers to constant, and constant pointers to constants
In this article, we will discuss the differences between constant pointer, pointers to constant & constant pointers to constants. Pointers are the variables that hold the address of some other variables, constants, or functions. There are several ways to qualify pointers using const. Pointers to
3 min read