Algorithm is a step-by-step procedure for solving a problem or accomplishing a task. In the context of data structures and algorithms, it is a set of well-defined instructions for performing a specific computational task. Algorithms are fundamental to computer science and play a very important role in designing efficient solutions for various problems. Understanding algorithms is essential for anyone interested in mastering data structures and algorithms.
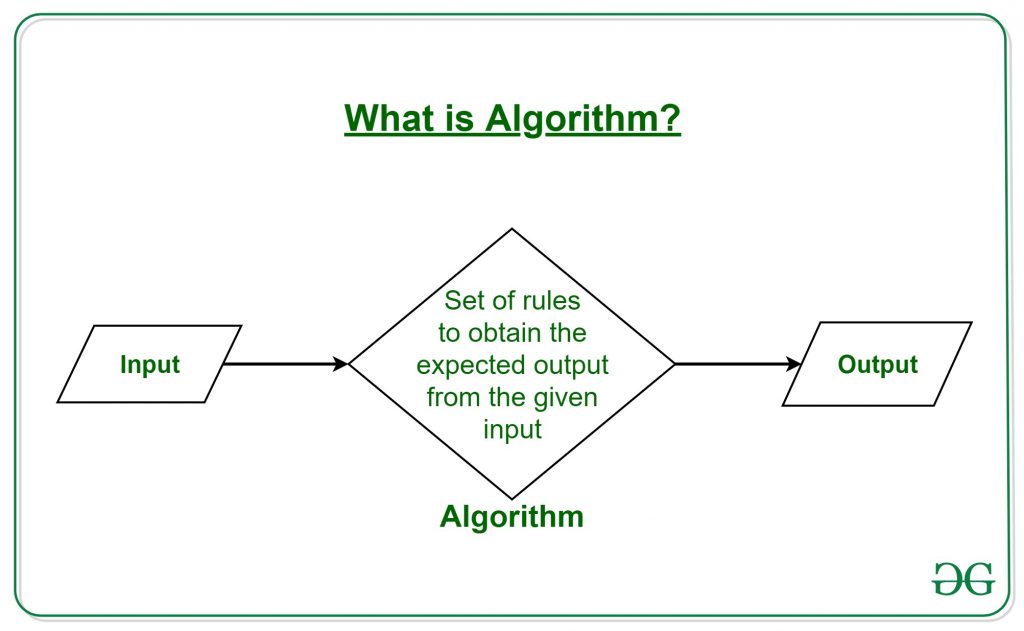
What is an Algorithm?
An algorithm is a finite sequence of well-defined instructions that can be used to solve a computational problem. It provides a step-by-step procedure that convert an input into a desired output.
Algorithms typically follow a logical structure:
- Input: The algorithm receives input data.
- Processing: The algorithm performs a series of operations on the input data.
- Output: The algorithm produces the desired output.
What is the Need for Algorithms?
Algorithms are essential for solving complex computational problems efficiently and effectively. They provide a systematic approach to:
- Solving problems: Algorithms break down problems into smaller, manageable steps.
- Optimizing solutions: Algorithms find the best or near-optimal solutions to problems.
- Automating tasks: Algorithms can automate repetitive or complex tasks, saving time and effort.
1. Analysis of Algorithms
Analysis of Algorithms is the process of evaluating the efficiency of algorithms, focusing mainly on the time and space complexity. This helps in evaluating how the algorithm's running time or space requirements grow as the size of input increases.
2. Mathematical Algorithms
Mathematical algorithms are used for analyzing and optimizing data structures and algorithms. Knowing basic concepts like divisibility, LCM, GCD, etc. can really help you understand how data structures work and improve your ability to design efficient algorithms.
3. Bitwise Algorithms
Bitwise algorithms are algorithms that operate on individual bits of numbers. These algorithms manipulate the binary representation of numbers like shifting bits, setting or clearing specific bits of a number and perform bitwise operations (AND, OR, XOR). Bitwise algorithms are commonly used in low-level programming, cryptography, and optimization tasks where efficient manipulation of individual bits is required.
4. Searching Algorithms
Searching Algorithms are used to find a specific element or item in a collection of data. These algorithms are widely used to retrieve data efficiently from large datasets.
5. Sorting Algorithms
Sorting algorithms are used to arrange the elements of a list in a specific order, such as numerical or alphabetical. It organizes the items in a systematic way, making it easier to search for and access specific elements.
6. Recursion
Recursion is a programming technique where a function calls itself within its own definition. It is usually used to solve problems that can be broken down into smaller instances of the same problem.
7. Backtracking Algorithm
Backtracking Algorithm is derived from the Recursion algorithm, with the option to revert if a recursive solution fails, i.e. in case a solution fails, the program traces back to the moment where it failed and builds on another solution. So basically it tries out all the possible solutions and finds the correct one.
8. Divide and Conquer Algorithm
Divide and conquer algorithms follow a recursive strategy to solve problems by dividing them into smaller subproblems, solving those subproblems, and combining the solutions to obtain the final solution.
9. Greedy Algorithm
Greedy Algorithm builds up the solution one piece at a time and chooses the next piece which gives the most obvious and immediate benefit i.e., which is the most optimal choice at that moment. So the problems where choosing locally optimal also leads to the global solutions are best fit for Greedy.
10. Dynamic Programming
Dynamic Programming is a method used to solve complex problems by breaking them down into simpler subproblems. By solving each subproblem only once and storing the results, it avoids redundant computations, leading to more efficient solutions for a wide range of problems.
11. Graph Algorithms
Graph algorithms are a set of techniques and methods used to solve problems related to graphs, which are a collection of nodes and edges. These algorithms perform various operations on graphs, such as searching, traversing, finding the shortest path, and determining connectivity. They are essential for solving a wide range of real-world problems, including network routing, social network analysis, and resource allocation.
12. Pattern Searching
Pattern Searching is a fundamental technique in DSA used to find occurrences of a specific pattern within a larger text. The Pattern Searching Algorithms use techniques like preprocessing to minimize unnecessary comparisons, making the search faster.
13. Branch and Bound Algorithm
Branch and Bound Algorithm is a method used in combinatorial optimization problems to systematically search for the best solution. It works by dividing the problem into smaller subproblems, or branches, and then eliminating certain branches based on bounds on the optimal solution. This process continues until the best solution is found or all branches have been explored.
14. Geometric Algorithms
Geometric algorithms are a set of algorithms that solve problems related to shapes, points, lines and polygons. Geometric algorithms are essential for solving a wide range of problems in computer science, such as intersection detection, convex hull computation, etc.
15. Randomized Algorithms
Randomized algorithms are algorithms that use randomness to solve problems. They make use of random input to achieve their goals, often leading to simpler and more efficient solutions. These algorithms may not product same result but are particularly useful in situations when a probabilistic approach is acceptable.
Related Articles: