Hospital Management Application using MERN Stack
Last Updated :
05 Aug, 2024
In the fast-paced world of healthcare, it's vital to manage hospital tasks effectively to ensure top-notch patient care. This article explores creating a strong Hospital Management App using the MERN stack – that's MongoDB, Express, React, and Node.js, breaking down the process for easier understanding.
Preview of final output: Let us have a look at how the final application will look like.
Final PreviewPrerequisite:
Steps to Setup Backend with Node and Express:
Step 1: Creating express app:
npm init -y
Step 2: Installing the required packages
npm install express mongoose body-parser
Project Structure:
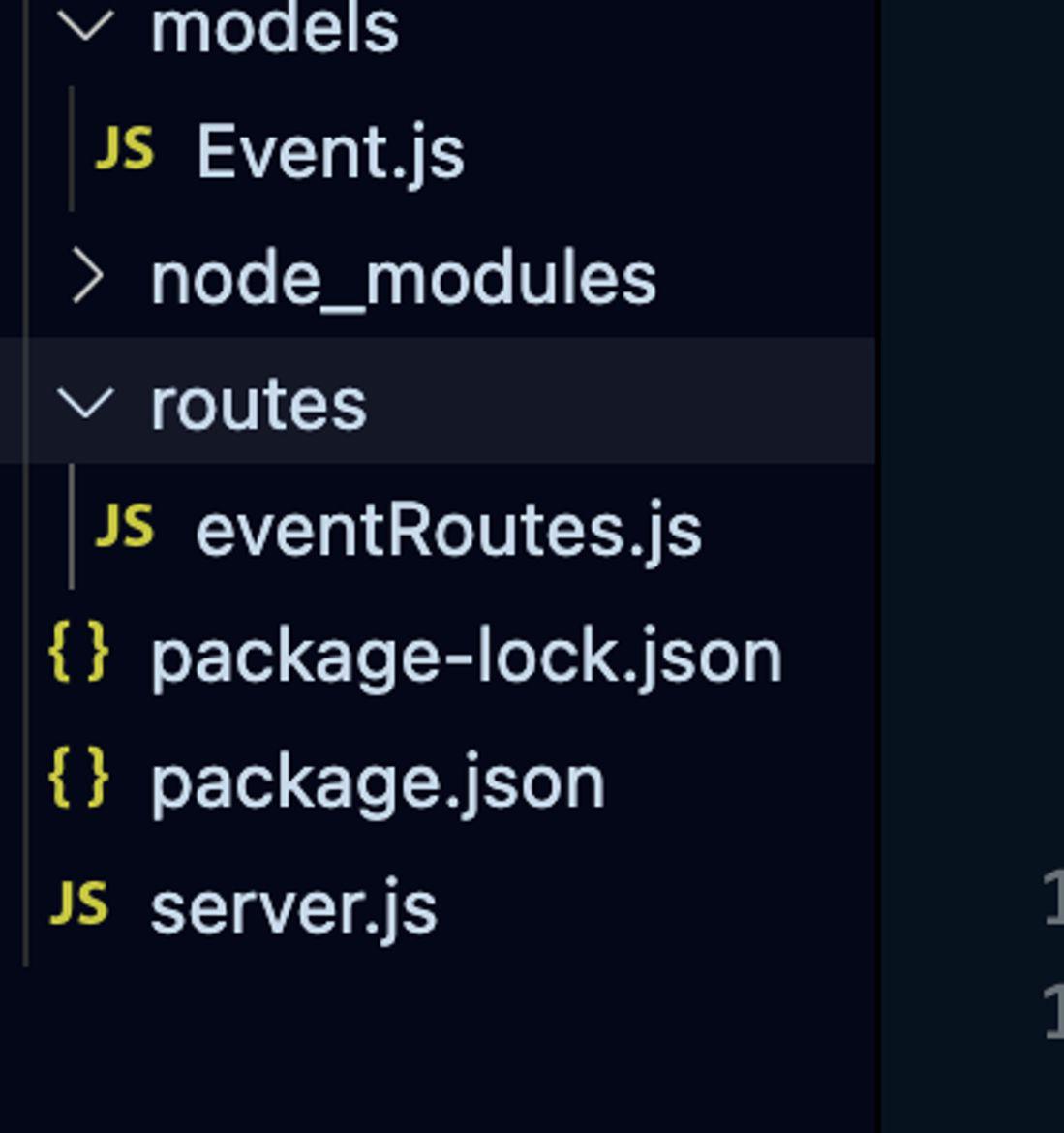
The updated dependencies in package.json file for backend will look like:
"dependencies": {
"body-parser": "^1.20.2",
"express": "^4.18.2",
"mongoose": "^8.0.0",
}
Approach to create backend:
- Create MongoDB Models:
- Design MongoDB models for `Appointment`, `Doctor`, and `Patient` in separate files (`Appointment.js`, `Doctor.js`, `Patient.js`).
- Set Up Express Routes:
- Create separate routes for appointments, doctors, and patients (`appointments.js`, `doctors.js`, `patients.js`).
- Implement CRUD (Create, Read, Update, Delete) operations for each resource.
- Connect to MongoDB:
- In your main `server.js`, connect to MongoDB using Mongoose.
- Don't forget to handle connection errors.
Example: Below is the code for the all the above files named above:
JavaScript
// server.js
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const bodyParser = require('body-parser');
const patientsRouter = require('./routes/patients');
const doctorsRouter = require('./routes/doctors');
const appoinmentsRouter = require('./routes/appointments')
const app = express();
const PORT = process.env.PORT || 5000;
app.use(cors());
app.use(bodyParser.json());
// Connect to MongoDB
mongoose.connect(
'mongodb://localhost:27017/hospital',
{
useNewUrlParser: true,
useUnifiedTopology: true
});
const connection = mongoose.connection;
connection.once('open', () => {
console.log('MongoDB database connection established successfully');
});
app.use('/patients', patientsRouter);
app.use('/doctors', doctorsRouter);
app.use('/appointments', appoinmentsRouter)
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
JavaScript
// routes/appointments.js
const express = require('express');
const router = express.Router();
const Appointment =
require('../models/Appointment');
// Get all appointments
router.route('/').get((req, res) => {
Appointment.find()
.then(appointments =>
res.json(appointments))
.catch(err =>
res.status(400).json('Error: ' + err));
});
// Add new appointment
router.route('/add').post((req, res) => {
const { patientName, doctorName, date } = req.body;
const newAppointment =
new Appointment({ patientName, doctorName, date });
newAppointment.save()
.then(savedAppointment => res.json(savedAppointment))
.catch(err => res.status(400).json('Error: ' + err));
});
// Update appointment data
router.route('/update/:id').post((req, res) => {
Appointment.findById(req.params.id)
.then(appointment => {
appointment.patientName =
req.body.patientName;
appointment.doctorName =
req.body.doctorName;
appointment.date =
req.body.date;
appointment.save()
.then(
() =>
res.json('Appointment updated!'))
.catch(
err => res.status(400)
.json('Error: ' + err));
})
.catch(
err => res.status(400)
.json('Error: ' + err));
});
// Delete appointment
router.route('/delete/:id')
.delete((req, res) => {
Appointment.findByIdAndDelete(req.params.id)
.then(
() => res
.json('Appointment deleted.'))
.catch(
err => res
.status(400).json('Error: ' + err));
});
module.exports = router;
JavaScript
// routes/doctors.js
const express = require('express');
const router = express.Router();
const Doctor = require('../models/Doctor');
// Get all doctors
router.route('/').get((req, res) => {
Doctor.find()
.then(doctors =>
res.json(doctors))
.catch(err =>
res.status(400)
.json('Error: ' + err));
});
// Add new doctor
router.route('/add')
.post((req, res) => {
const { name, specialty } = req.body;
const newDoctor =
new Doctor({ name, specialty });
newDoctor.save()
// Return the savedDoctor object
.then(savedDoctor =>
res.json(savedDoctor))
.catch(
err =>
res.status(400)
.json('Error: ' + err));
});
// Update doctor data
router.route('/update/:id')
.post((req, res) => {
Doctor.findById(req.params.id)
.then(doctor => {
if (!doctor) {
return res.status(404)
.json('Doctor not found');
}
doctor.name = req.body.name;
doctor.specialty = req.body.specialty;
doctor.save()
.then(() => res.json('Doctor updated!'))
.catch(err => res.status(400)
.json('Error: ' + err));
})
.catch(err => res.status(400)
.json('Error: ' + err));
});
// Delete doctor by ID
router.route('/delete/:id').delete((req, res) => {
Doctor.findByIdAndDelete(req.params.id)
.then(doctor => {
if (!doctor) {
return res.status(404)
.json('Doctor not found');
}
res.json('Doctor deleted!');
})
.catch(err => res.status(400)
.json('Error: ' + err));
});
module.exports = router;
JavaScript
// routes/patients.js
const express = require('express');
const router = express.Router();
const Patient = require('../models/Patient');
// Get all patients
router.route('/').get((req, res) => {
Patient.find()
.then(patients =>
res.json(patients))
.catch(err =>
res.status(400)
.json('Error: ' + err));
});
// Add new patient
router.route('/add')
.post((req, res) => {
const { name, age, gender } = req.body;
const newPatient =
new Patient({ name, age, gender });
newPatient.save()
.then(savedPatient =>
res.json(savedPatient))
.catch(err => res.status(400)
.json('Error: ' + err));
});
// Update patient data
router.route('/update/:id')
.post((req, res) => {
console.log('hihhhhiuhiihihiuhiuh');
Patient.findById(req.params.id)
.then(patient => {
if (!patient) {
return res.status(404)
.json('Patient not found');
}
patient.name = req.body.name;
patient.age = req.body.age;
patient.gender = req.body.gender;
patient.save()
.then(() => res.json('Patient updated!'))
.catch(err => res.status(400)
.json('Error: ' + err));
})
.catch(err => res.status(400)
.json('Error: ' + err));
});
// Delete patient by ID
router.route('/delete/:id')
.delete((req, res) => {
Patient.findByIdAndDelete(req.params.id)
.then(patient => {
if (!patient) {
return res.status(404)
.json('Patient not found');
}
res.json('Patient deleted!');
})
.catch(err => res.status(400)
.json('Error: ' + err));
});
module.exports = router;
JavaScript
// models/Appointment.js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const appointmentSchema = new Schema({
patientName: { type: String, required: true },
doctorName: { type: String, required: true },
date: { type: Date, required: true },
// Add more fields as needed
});
const Appointment =
mongoose.model('Appointment', appointmentSchema);
module.exports = Appointment;
JavaScript
// models/Doctor.js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const doctorSchema = new Schema({
name: { type: String, required: true },
specialty: { type: String, required: true },
// Add more fields as needed
});
const Doctor =
mongoose.model('Doctor', doctorSchema);
module.exports = Doctor;
JavaScript
// models/Patient.js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const patientSchema = new Schema({
name: { type: String, required: true },
age: { type: Number, required: true },
gender: { type: String, required: true },
// Add more fields as needed
});
const Patient = mongoose.model('Patient', patientSchema);
module.exports = Patient;
Steps to Setup Frontend with React
Step 1: Create React App:
npx create-react-app myapp
Step 2: Switch to the project directory:
cd myapp
Step 3: Installing the required packages:
npm install axios react-router-dom
Project Structure:
Project StructureThe updated dependencies in package.json for frontend will look like:
"dependencies": {
"axios": "^1.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.17.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Approach to create Frontend:
- Create Components for `Appointments`, `Doctors`, `Patients`, `AppointmentCard`, `DoctorCard`, and `PatientCard` within `src/components`
- Create a main `App` component that utilizes React Router for navigation.
- Design styles for each component to enhance the visual appeal.
Example: Below is the code for the all the above files named above:
CSS
/* App.css */
.container {
max-width: 90vw;
margin: 0 auto;
padding: 20px 150px;
background-color: #F5EEE6;
}
nav {
background-color: #00a7aa;
padding: 10px;
border-radius: 10px;
}
ul {
list-style: none;
padding: 0;
margin: 0;
display: flex;
}
li {
margin-right: 20px;
}
a {
text-decoration: none;
color: white;
font-weight: bold;
}
a:hover {
color: #ffd700;
}
.active {
color: #ffd700;
}
/* FLEX */
.flex-column {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.flex-row {
display: flex;
flex-wrap: wrap;
flex-direction: row;
justify-content: space-between;
align-items: center;
}
CSS
/* /src/components/App */
/* Appointment card */
.appointment-card {
border: 1px solid #ddd;
border-radius: 8px;
width: 300px;
padding: 16px;
margin: 16px 0;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
transition: box-shadow 0.3s ease;
&:hover {
box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
}
p {
font-size: 16px;
margin-bottom: 10px;
}
p {
span {
font-weight: 900;
}
}
button {
background-color: #007bff;
color: #fff;
border: none;
padding: 8px 16px;
margin-right: 8px;
cursor: pointer;
transition: background-color 0.3s ease;
&:hover {
background-color: #002e5f;
}
}
}
/* Add this to a new CSS file, e.g., AppointmentForm.css */
.appointment-form {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 8px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.appointment-form h4 {
font-size: 24px;
margin-bottom: 20px;
}
.appointment-form label {
display: block;
margin-bottom: 8px;
}
.appointment-form input {
width: 100%;
padding: 8px;
margin-bottom: 16px;
border: 1px solid #ddd;
border-radius: 4px;
}
.appointment-form button {
background-color: #007bff;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 4px;
cursor: pointer;
transition: background-color 0.3s ease;
&:hover {
background-color: #0056b3;
}
}
.appointments {
display: flex;
flex-direction: column;
align-items: center;
height: 100vh;
width: 30vw;
}
.add-form {
display: flex;
flex-direction: column;
align-items: center;
margin-bottom: 250px;
}
CSS
/* /src/components/Doctors.css */
.main-doc-container {
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
}
.doctors-section {
width: 45%;
height: 100vh;
text-align: center;
margin-left: 16px;
/* Added margin for spacing */
}
/* Add the doctor card styles */
.doctor-card {
background-color: #fff;
border: 1px solid #ddd;
border-radius: 8px;
padding: 16px;
margin-bottom: 16px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease-in-out;
&:hover {
transform: scale(1.02);
}
p {
margin: 0;
}
button {
margin-top: 8px;
cursor: pointer;
}
}
/* Add the form section styles */
.form-sections {
background-color: #fff;
border: 1px solid #ddd;
border-radius: 8px;
padding: 16px;
width: 45%;
margin-bottom: 306px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
h4 {
margin-bottom: 16px;
text-align: center;
}
form {
display: flex;
flex-direction: column;
label {
margin-bottom: 8px;
}
input {
padding: 8px;
margin-bottom: 16px;
}
button {
align-self: flex-start;
padding: 8px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
&:hover {
background-color: #0056b3;
}
}
}
}
button {
margin-top: 8px;
cursor: pointer;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
padding: 8px;
cursor: pointer;
&:hover {
background-color: #0056b3;
}
}
.btn-container {
display: flex;
justify-content: space-between;
width: 100%;
}
CSS
/* /src/components/Patients.css */
.patient-main {
display: flex;
}
.form-sections {
width: 45%;
margin-right: 20px;
height: 400px;
}
.patients-section {
width: 45%;
}
.patient-card {
background-color: #fff;
border: 1px solid #ddd;
border-radius: 8px;
padding: 16px;
margin-bottom: 16px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease-in-out;
&:hover {
transform: scale(1.02);
}
h4 {
margin-bottom: 8px;
}
p {
margin: 8px 0;
}
button {
margin-top: 8px;
cursor: pointer;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
padding: 8px;
cursor: pointer;
&:hover {
background-color: #0056b3;
}
}
}/* /src/components/Patients.css */
.patient-main {
display: flex;
}
.form-sections {
width: 45%;
margin-right: 20px;
height: 400px;
}
.patients-section {
width: 45%;
}
.patient-card {
background-color: #fff;
border: 1px solid #ddd;
border-radius: 8px;
padding: 16px;
margin-bottom: 16px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease-in-out;
&:hover {
transform: scale(1.02);
}
h4 {
margin-bottom: 8px;
}
p {
margin: 8px 0;
}
button {
margin-top: 8px;
cursor: pointer;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
padding: 8px;
cursor: pointer;
&:hover {
background-color: #0056b3;
}
}
}
JavaScript
//App.js
import React from 'react';
import {
BrowserRouter as Router,
Routes, Route,
Link, useNavigate
} from 'react-router-dom';
import Appointments
from './components/Appointments';
import Doctors from './components/Doctors';
import Patients from './components/Patients';
import './App.css'
const App = () => {
const isLinkActive =
(path) =>
window.location.pathname === path;
return (
<Router>
<div className="container">
<h1 style={{ color: "green" }}>
GFG- Hospital Managment App
</h1>
<nav>
<ul>
<li className={
isLinkActive('/appointments')
? 'active' : ''}>
<Link to="/appointments">
Appointments
</Link>
</li>
<li className={
isLinkActive('/doctors') ?
'active' : ''}>
<Link to="/doctors">
Doctors
</Link>
</li>
<li className={
isLinkActive('/patients') ?
'active' : ''}>
<Link to="/patients">
Patients
</Link>
</li>
</ul>
</nav>
<Routes>
<Route path="/appointments"
element={<Appointments />} />
<Route path="/"
element={<Appointments />} />
<Route path="/doctors"
element={<Doctors />} />
<Route path="/patients"
element={<Patients />} />
</Routes>
</div>
</Router>
);
}
export default App;
JavaScript
//Appointments.js
import React,
{
useState,
useEffect
} from 'react';
import axios from 'axios';
import AppointmentCard
from './AppointmentCard';
import './Appointment.css'
const Appointments = () => {
const [appointments, setAppointments] = useState([]);
const [newAppointment, setNewAppointment] =
useState(
{
patientName: '',
doctorName: '', date: ''
});
const [selectedAppointment, setSelectedAppointment] = useState(null);
const [isEditMode, setIsEditMode] = useState(false);
useEffect(() => {
axios
.get(
'http://localhost:5000/appointments')
.then(
response =>
setAppointments(response.data))
.catch(
error =>
console.error('Error fetching appointments:', error)
);
}, []);
const handleAddAppointment =
(e) => {
e.preventDefault();
axios
.post(
'http://localhost:5000/appointments/add', newAppointment)
.then(response => {
console.log(response.data);
setAppointments(
[
...appointments,
response.data]);
setNewAppointment(
{
patientName: '',
doctorName: '', date: ''
});
})
.catch(error =>
console.error('Error adding appointment:', error));
};
const handleUpdateAppointment =
(id, e) => {
e.preventDefault();
axios
.post(
`http://localhost:5000/appointments/update/${id}`, selectedAppointment)
.then(response => {
console.log(response.data);
const updateApp = {
...selectedAppointment,
_id: id
};
setAppointments(
appointments.map(
appointment =>
(appointment._id === id
? updateApp :
appointment)
));
setSelectedAppointment(null);
setIsEditMode(false); // Switch back to Add mode
})
.catch(
error =>
console.error('Error updating appointment:', error));
};
const handleDeleteAppointment =
(id) => {
axios
.delete(
`http://localhost:5000/appointments/delete/${id}`)
.then(response => {
console.log(response.data);
setAppointments(
appointments.filter(
appointment =>
appointment._id !== id)
);
})
.catch(error =>
console.error('Error deleting appointment:', error));
};
const handleEditAppointment =
(appointment) => {
setSelectedAppointment(appointment);
setIsEditMode(true); // Switch to Edit mode
};
return (
<div className='flex-row' style={{ width: "100%" }}>
<div className='flex-column'>
<div className='add-form'>
<h4>
{
isEditMode ?
'Edit Appointment' :
'Add New Appointment'
}
</h4>
<form className="appointment-form"
onSubmit={
isEditMode ?
(e) =>
handleUpdateAppointment(selectedAppointment._id, e) :
handleAddAppointment
}>
<label>Patient Name:</label>
<input type="text"
value={
isEditMode ?
selectedAppointment.patientName :
newAppointment.patientName
}
onChange={
(e) =>
isEditMode ?
setSelectedAppointment(
{
...selectedAppointment,
patientName: e.target.value
}) :
setNewAppointment(
{
...newAppointment,
patientName: e.target.value
})} />
<label>Doctor Name:</label>
<input type="text"
value={
isEditMode ?
selectedAppointment.doctorName :
newAppointment.doctorName
}
onChange={
(e) =>
isEditMode ?
setSelectedAppointment(
{
...selectedAppointment,
doctorName: e.target.value
}) :
setNewAppointment(
{
...newAppointment,
doctorName: e.target.value
})} />
<label>Date:</label>
<input type="date"
value={
isEditMode ?
selectedAppointment.date :
newAppointment.date
}
onChange={
(e) =>
isEditMode ?
setSelectedAppointment(
{
...selectedAppointment,
date: e.target.value
}) :
setNewAppointment(
{
...newAppointment,
date: e.target.value
})} />
<button type="submit">
{
isEditMode ?
'Update Appointment' :
'Add Appointment'
}
</button>
</form>
</div>
</div>
<div className='appointments'>
<h3>Appointments
(
{
appointments.length
})
</h3>
<div className="appointment-list">
{appointments.map(appointment => (
<AppointmentCard
key={appointment._id}
appointment={appointment}
onEdit={handleEditAppointment}
onDelete={handleDeleteAppointment}
/>
))}
</div>
</div>
</div>
);
};
export default Appointments;
JavaScript
// src/components/AppointmentCard.js
import React from 'react';
const AppointmentCard =
(
{
appointment,
onEdit,
onDelete
}
) => {
return (
<div
className="appointment-card">
<p>
<span>
Patient:
</span>
{appointment.patientName}
</p>
<p>
<span>
Doctor:
</span>
{appointment.doctorName}</p>
<p>
<span>
Date:
</span>
{
new Date(appointment.date)
.toLocaleDateString()
}
</p>
<div className='btn-container'>
<button onClick={
() =>
onEdit(appointment)
}>
Edit
</button>
<button onClick={
() =>
onDelete(appointment._id)
}>
Delete
</button>
</div>
</div>
);
};
export default AppointmentCard;
JavaScript
//Doctors.js
import React,
{
useState,
useEffect
} from 'react';
import axios from 'axios';
import DoctorCard from './DoctorCard';
import './Doctors.css'
const Doctors = () => {
const [doctors, setDoctors] = useState([]);
const [newDoctor, setNewDoctor] =
useState(
{
name: '',
specialty: ''
});
const [selectedDoctor, setSelectedDoctor] = useState(null);
const [isEditMode, setIsEditMode] = useState(false);
useEffect(
() => {
axios
.get('http://localhost:5000/doctors')
.then(
response =>
setDoctors(response.data))
.catch(
error =>
console.error('Error fetching doctors:', error)
);
}, []);
const handleAddDoctor =
(e) => {
e.preventDefault();
axios
.post(
'http://localhost:5000/doctors/add', newDoctor)
.then(
response => {
console.log("doc", response.data);
setDoctors(
[
...doctors,
response.data
]
);
setNewDoctor(
{
name: '',
specialty: ''
});
})
.catch(
error =>
console.error('Error adding doctor:', error));
};
const handleUpdateDoctor =
(id, e) => {
e.preventDefault();
axios
.post(
`http://localhost:5000/doctors/update/${id}`, selectedDoctor)
.then(response => {
const updateDoc = {
...selectedDoctor,
_id: id
};
console.log('update doc', updateDoc);
setDoctors(
doctors.map(
doctor =>
(doctor._id === id ? updateDoc : doctor)));
setSelectedDoctor(null);
setIsEditMode(false); // Switch back to Add mode
})
.catch(
error =>
console.error('Error updating doctor:', error));
};
const handleDeleteDoctor = (id) => {
axios.delete(
`http://localhost:5000/doctors/delete/${id}`)
.then(response => {
console.log(response.data);
setDoctors(
doctors
.filter(doctor => doctor._id !== id)
);
})
.catch(
error =>
console.error('Error deleting doctor:', error));
};
const handleEditDoctor =
(doctor) => {
setSelectedDoctor(doctor);
setIsEditMode(true); // Switch to Edit mode
};
return (
<div className='main-doc-container '>
<div className='form-sections '>
<h4>
{
isEditMode ?
'Edit Doctor' :
'Add New Doctor'
}
</h4>
<form
onSubmit={
isEditMode ?
(e) =>
handleUpdateDoctor(selectedDoctor._id, e) :
handleAddDoctor}>
<label>Name: </label>
<input
type="text"
value={
isEditMode ?
selectedDoctor.name :
newDoctor.name
}
onChange={
(e) =>
isEditMode ?
setSelectedDoctor(
{
...selectedDoctor,
name: e.target.value
}) :
setNewDoctor(
{
...newDoctor,
name: e.target.value
})} />
<br />
<label>Specialty: </label>
<input type="text"
value=
{
isEditMode ?
selectedDoctor.specialty :
newDoctor.specialty
}
onChange={
(e) =>
isEditMode ?
setSelectedDoctor(
{
...selectedDoctor,
specialty: e.target.value
}
) :
setNewDoctor(
{
...newDoctor,
specialty: e.target.value
}
)} />
<br />
<button type="submit">
{
isEditMode ?
'Update Doctor' :
'Add Doctor'
}</button>
</form>
</div>
<div className='doctors-section '>
<h3>Doctors({doctors.length}) </h3>
<div className="doctor-list">
{doctors.map(doctor => (
<DoctorCard
key={doctor._id}
doctor={doctor}
onEdit={handleEditDoctor}
onDelete={handleDeleteDoctor}
/>
))}
</div>
</div>
</div>
);
};
export default Doctors;
JavaScript
// src/components/DoctorCard.js
import React from 'react';
const DoctorCard =
(
{
doctor, onEdit,
onDelete
}
) => {
return (
<div className="doctor-card">
<p>
{doctor.name} -
{doctor.specialty}
</p>
<div className='btn-container'>
<button onClick={
() =>
onEdit(doctor)
}>
Edit
</button>
<button onClick={
() =>
onDelete(doctor._id)
}>
Delete
</button>
</div>
</div>
);
};
export default DoctorCard;
JavaScript
// src/components/Patients.js
import React, { useState, useEffect } from 'react';
import axios from 'axios';
import './Patients.css';
import PatientCard from './PatientCard';
const Patients = () => {
const [patients, setPatients] = useState([]);
const [newPatient, setNewPatient] =
useState({ name: '', age: '', gender: '' });
const [selectedPatient, setSelectedPatient] = useState(null);
const [isEditMode, setIsEditMode] = useState(false);
useEffect(
() => {
axios.get('http://localhost:5000/patients')
.then(response => setPatients(response.data))
.catch(error =>
console.error('Error fetching patients:', error));
}, []);
const handleAddPatient =
(e) => {
e.preventDefault();
axios.post(
'http://localhost:5000/patients/add', newPatient)
.then(response => {
console.log(response.data);
setPatients([...patients, response.data]);
setNewPatient({ name: '', age: '', gender: '' });
})
.catch(error =>
console.error('Error adding patient:', error));
};
const handleUpdatePatient =
(id, e) => {
e.preventDefault();
axios.post(
`http://localhost:5000/patients/update/${id}`, selectedPatient)
.then(response => {
const updatePat = {
...selectedPatient,
_id: id
};
console.log('update patient', updatePat);
setPatients(
patients.map(
patient =>
(patient._id === id
? updatePat : patient)));
setSelectedPatient(null);
setIsEditMode(false); // Switch back to Add mode
})
.catch(
error =>
console.error('Error updating patient:', error));
};
const handleDeletePatient =
(id) => {
axios.delete(
`http://localhost:5000/patients/delete/${id}`)
.then(response => {
console.log(response.data);
setSelectedPatient(null);
setPatients(
patients.filter(
patient => patient._id !== id));
})
.catch(
error =>
console.error('Error deleting patient:', error));
};
const handleEditPatient =
(patient) => {
setSelectedPatient(patient);
setIsEditMode(true); // Switch to Edit mode
};
return (
<div className='patient-main '>
<div className='form-sections '>
<h4>
{
isEditMode ?
'Edit Patient' :
'Add New Patient'
}
</h4>
<form onSubmit=
{
isEditMode ?
(e) =>
handleUpdatePatient(selectedPatient._id, e) :
handleAddPatient}>
<label>Name: </label>
<input type="text"
value={
isEditMode ?
selectedPatient.name :
newPatient.name
}
onChange={
(e) =>
isEditMode
? setSelectedPatient(
{
...selectedPatient,
name: e.target.value
}) :
setNewPatient(
{
...newPatient,
name: e.target.value
}
)} />
<br />
<label>Age: </label>
<input type="text"
value=
{
isEditMode ?
selectedPatient.age : newPatient.age
}
onChange={
(e) =>
isEditMode ?
setSelectedPatient(
{
...selectedPatient,
age: e.target.value
}) :
setNewPatient(
{
...newPatient,
age: e.target.value
}
)} />
<br />
<label>Gender: </label>
<input type="text"
value=
{
isEditMode ?
selectedPatient.gender :
newPatient.gender
} onChange={
(e) =>
isEditMode ?
setSelectedPatient(
{
...selectedPatient,
gender: e.target.value
}) :
setNewPatient(
{
...newPatient,
gender: e.target.value
})} />
<br />
<button type="submit">
{
isEditMode ?
'Update Patient' :
'Add Patient'
}
</button>
</form>
</div>
<div className='patients-section '>
<h3 style={{ textAlign: "center" }}>
Patients
({patients.length})
</h3>
<div className="patient-list">
{patients.map(patient => (
<PatientCard
key={patient._id}
patient={patient}
onEdit={handleEditPatient}
onDelete={handleDeletePatient}
/>
))}
</div>
</div>
</div>
);
};
export default Patients;
JavaScript
import React from 'react';
const PatientCard =
(
{
patient, onEdit,
onDelete
}
) => {
return (
<div className="patient-card">
<h4>{patient.name}</h4>
<p>Age: {patient.age}</p>
<p>Gender: {patient.gender}</p>
<div className='btn-container'
style={{ width: "100%" }}>
<button onClick={
() =>
onEdit(patient)}>
Edit
</button>
<button onClick={
() =>
onDelete(patient._id)
}>
Delete
</button>
</div>
</div>
);
};
export default PatientCard;
Steps to run the App:
To run server.js:
node server.js
To run frontend:
npm start
Output:
Data Saved in Db:
Data in Db
Similar Reads
35+ MERN Stack Projects with Source Code [2024]
The MERN stack, comprising MongoDB, Express JS, React, and Node JS, is a powerful combination that enables developers to build full-stack web applications using JavaScript. MongoDB serves as the database, Express.js handles server-side logic, React manages the client-side interface, and Node.js faci
6 min read
Stock Market Portfolio App using MERN Stack
The Stock Market Portfolio project is a web application that helps to efficiently manage and track stock market investments and portfolios. In this article, we will see a step-wise process of building a Stock Market Portfolio using the power of the MERN stack. Project Preview: Let us have a look at
5 min read
Hospital Management Application using MERN Stack
In the fast-paced world of healthcare, it's vital to manage hospital tasks effectively to ensure top-notch patient care. This article explores creating a strong Hospital Management App using the MERN stack â that's MongoDB, Express, React, and Node.js, breaking down the process for easier understand
15+ min read
Social Media Platform using MERN Stack
In web development, creating a "Social Media Website" will showcase and utilising the power of MERN stack â MongoDB, Express, React, and Node. This application will provide users the functionality to add a post, like the post and able to comment on it. Preview Image: Let us have a look at how the fi
7 min read
Bookstore Ecommerce App using MERN Stack
Bookstore E-commerce project is a great way to showcase your understanding of full-stack development. In this article, we'll walk through the step-by-step process of creating a Bookstore E-commerce using the MERN (MongoDB, Express.js, React, Node.js) stack. This project will showcase how to set up a
8 min read
Chat Website using MERN Stack
The "Chat Website" project is a dynamic web application that is used for real-time communication. The MERN stack, comprised of MongoDB, Express.js, React.js, and Node.js, is a powerful combination of technologies for developing robust and scalable web applications. In this article, we'll explore the
4 min read
Restaurant App using MERN Stack
Creating a Restaurant app will cover a lot of features of the MERN stack. In this tutorial, we'll guide you through the process of creating a restaurant application using the MERN stack. The application will allow users to browse through a list of restaurants, view their menus, and add items to a sh
11 min read
Real Estate Management using MERN
In this article, we will guide you through the process of building a Real Estate Management Application using the MERN stack. MERN stands for MongoDB, Express, React, and Node. MongoDB will serve as our database, Express will handle the backend, React will create the frontend, and Node.js will be th
9 min read
Fruit and Vegetable Market Shop using MERN
In this comprehensive guide, we'll walk through the step-by-step process of creating a Fruit and Vegetable Market Shop using the MERN (MongoDB, Express.js, React, Node.js) stack. This project will showcase how to set up a full-stack web application where users can view, filter, and purchase various
8 min read
Event Management Web App using MERN
In this guide, we'll walk through the step-by-step process of building a feature-rich Event Management Web App. We will make use of the MERN stack to build this project. Preview of final output: Let us have a look at how the final output will look like. PrerequisiteReact JSMongoDBExpressNode JSAppro
9 min read