How to make div elements display inline using CSS ?
Last Updated :
16 Sep, 2024
Displaying div elements inline allows them to share horizontal space and appear in a single line, making your layout more dynamic and responsive. This guide explores different CSS techniques to achieve this, including flexbox, inline-block, float, and span elements.
Why Display Div Elements Inline?
- Responsive Layouts: Inline elements adapt to the width of the container, making them suitable for responsive design.
- Space Optimization: Placing elements in a row can save space and create a cleaner look.
- Improved User Experience: Inline elements can enhance the flow of information and the overall design of the webpage.
CSS Properties Used
In this article, we will explore the following CSS properties to display div elements inline:
- Display: Using display: flex and display: inline-block properties.
- Float: Using float: left to position elements side by side.
- Span Elements: Utilizing span tags, which render inline by default.
Approach 1: Using Flexbox (display: flex)
In this approach, we have set the display: flex and flex-direction: row to the parent div of all the div elements. Due to the flex-direction: row property all the elements inside the parent div will be shown in a single row.
Example: In this example, we are using the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<style>
.main {
display: flex;
flex-direction: row;
font-size: 30px;
color: green;
border: 4px dashed green;
padding: 5px;
width: 400px;
}
.main div {
border: 2px solid red;
margin: 10px 20px;
width: 100px;
}
</style>
</head>
<body>
<div class="main">
<div>Geeks</div>
<div>for</div>
<div>Geeks</div>
</div>
</body>
</html>
Output:
Users can see that all div elements inside the parent div are displaying inline.
Approach 2: Using Inline-Block (display: inline-block)
In this approach, we will apply the display: inline-block property to all the div we need to display inline. The display:inline-block property will set all div elements side by side.
Example: In this example, we are using the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<style>
div {
display: inline-block;
color: green;
border: 2px solid red;
margin: 10px 20px;
width: 120px;
font-size: 40px;
}
</style>
</head>
<body>
<div>Geeks</div>
<div>for</div>
<div>Geeks</div>
</body>
</html>
Output:
Users can see all div elements displayed inline.
Approach 3: Using Float (float: left or float: right)
In this approach, we will apply the float: left property to all the div elements to display them inline. Also, users can use the float: right CSS property to show all div elements in reverse order from the right side.
Example: In this example, we are using the above approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<style>
div {
float: left;
color: green;
border: 2px solid red;
margin: 10px 20px;
width: 120px;
font-size: 40px;
}
</style>
</head>
<body>
<div>Geeks</div>
<div>for</div>
<div>Geeks</div>
</body>
</html>
Output:
Users can see in the below output image how all div elements look when we apply the float: left CSS property to all div elements.
Approach 4: Using Span Elements
In this approach, we will use the span element instead of the div element. When the user needs to write only text inside the div tag, they can use the span tag as all span elements render inline by default.
Example: In this example, we are using the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<style>
span {
color: green;
border: 2px solid red;
margin: 10px 20px;
width: 100px;
font-size: 30px;
}
</style>
</head>
<body>
<span>Geeks</span>
<span>for</span>
<span>Geeks</span>
</body>
</html>
Output: In the below output image, users can see how the span element renders inline.
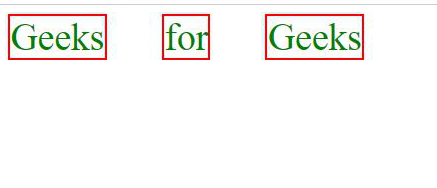
Displaying div elements inline can be achieved using various CSS techniques such as flexbox, inline-block, float, or even using span elements for text. Each approach offers different benefits and can be selected based on the specific needs of your layout.