How to Toggle an Element Class in JavaScript ?
Last Updated :
20 Sep, 2024
In JavaScript, toggling an element class refers to the process of dynamically adding or removing a CSS class from an HTML element. This allows developers to easily change an element’s appearance or behavior in response to user actions, such as clicks or events.
These are the following methods for toggling an element Class:
Method 1: Using the toggle() method
The toggle() method in JavaScript’s classList object adds a specified class to an element if it’s not present, and removes it if it is. This allows for easy, dynamic switching of styles or behaviors in response to user interactions or events.
Example: In this example we toggles a CSS class on a paragraph element. When the button is clicked, it dynamically adds or removes the “paragraphClass,” changing the font size and color of the text.
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Geeks for Geeks</title>
<style>
.paragraphClass {
font-size: 30px;
color: red;
}
#Button {
margin-top: 15px;
}
</style>
<script>
function myFunc() {
let para = document.getElementById("p");
para.classList.toggle("paragraphClass");
}
</script>
</head>
<body>
<p id="p">
Click on the button to toggle
between the class to see the
effects
</p>
<button id="Button" onclick="myFunc()">
Click Me
</button>
</body>
</html>
Output:
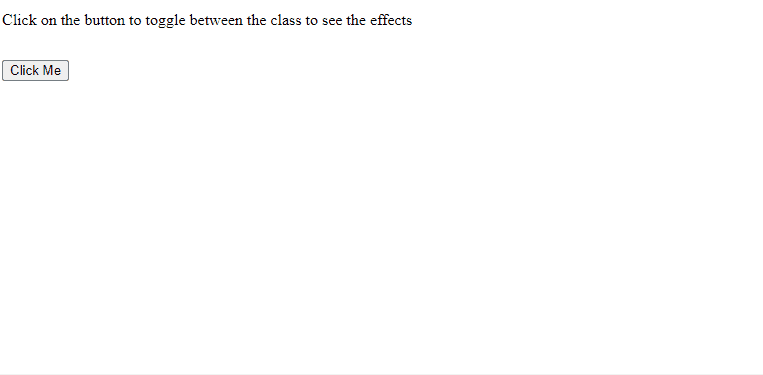
How to Toggle an Element Class in JavaScript ?
Method 2: Using contains(), add() and remove() Method
In this example we use classList.contains() to check if an element has a specific class, classList.add() to add a class, and classList.remove() to remove it. These methods offer fine control over element class manipulation dynamically.
Example: In this example we toggles a CSS class using contains(), add(), and remove(). It checks if the paragraph has a specific class, removes it if present, or adds it otherwise on button click.
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Geeks for Geeks</title>
<style>
.paragraphClass {
font-size: 30px;
color: red;
}
#Button {
margin-top: 15px;
}
</style>
<script>
function myFunc() {
let para = document.getElementById("p");
if (para.classList.contains("paragraphClass")) {
para.classList.remove("paragraphClass");
}
else {
para.classList.add("paragraphClass");
}
}
</script>
</head>
<body>
<p id="p">
Click on the button to toggle
between the class to see the
effects
</p>
<button id="Button" onclick="myFunc()">
Click Me
</button>
</body>
</html>
Output:
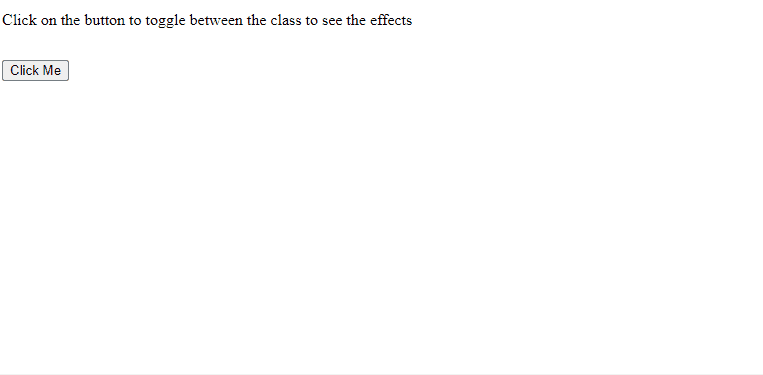
How to Toggle an Element Class in JavaScript ?