JavaScript Break Statement
Last Updated :
19 Nov, 2024
JavaScript break statement is used to terminate the execution of the loop or the switch statement when the condition is true.
In Switch Block (To come out of the block)
JavaScript
const fruit = "Mango";
switch (fruit) {
case "Apple":
console.log("Apple is healthy.");
break;
case "Mango":
console.log("Mango is delicious.");
break;
default:
console.log("No fruit chosen.");
}
OutputMango is delicious.
Why do we need to use break with Switch?
In the below example, the fruit name is apple but the given output is for the two cases. This is because of the break statement. In the case of Apple, we are not using a break statement which means the block will run for the next case also till the break statement not appear.
JavaScript
const fruit = "Apple";
switch (fruit) {
case "Apple":
console.log("Apple is healthy.");
case "Mango":
console.log("Mango is delicious.");
break;
default:
console.log("No fruit chosen.");
}
OutputApple is healthy.
Mango is delicious.
In a For Loop (To come out of the Loop)
JavaScript
for (let i = 1; i < 6; i++) {
if (i == 4) break;
console.log(i);
}
How does Break Work?
While and Do-While Loop Examples
JavaScript
// Using break in a while loop
let i = 1;
while (i <= 5) {
console.log(i);
if (i === 3) {
break;
}
i++;
}
// Using break in a do-while loop
let j = 1;
do {
console.log(j);
if (j === 3) {
break;
}
j++;
} while (j <= 5);
How does it Work?
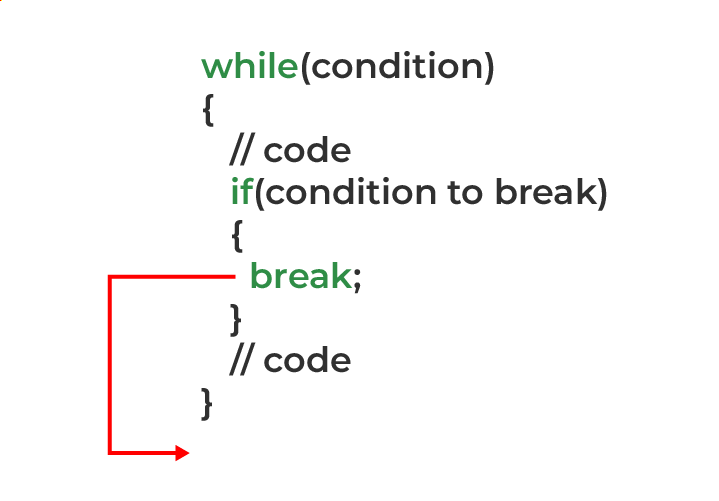
Break with Labels
In JavaScript, we can use a break
statement with a label to exit from a specific loop, even if it’s nested inside another loop. This is useful when you need to break out of a nested loop structure, and just using a simple break
would only exit the innermost loop.
JavaScript
outerLoop:
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
if (i === 1 && j === 1) {
break outerLoop;
}
console.log(`i: ${i}, j: ${j}`);
}
}
Outputi: 0, j: 0
i: 0, j: 1
i: 0, j: 2
i: 1, j: 0