Left Shift and Right Shift Operators in C/C++
Last Updated :
28 Nov, 2024
In C/C++, left shift (<<) and right shift (>>) operators are binary bitwise operators that are used to shift the bits either left or right of the first operand by the number of positions specified by the second operand allowing efficient data manipulation. In this article, we will learn about the left shift and right shift operators.
Let’s take a look at an example:
C++
#include <iostream>
using namespace std;
int main() {
// a = 21(00010101)
unsigned char a = 21;
// The result is 00101010
cout << "a << 1 = " << (a << 1) << endl;
// The result is 000001010
cout << "a >> 2 = " << (a >> 2);
return 0;
}
C
#include <stdio.h>
int main() {
// a = 21(00010101)
unsigned char a = 21;
// The result is 00101010
printf("a << 1 = %d\n", (a << 1));
// The result is 000001010
printf("a >> 2 = %d", (a >> 2));
return 0;
}
Outputa << 1 = 42
a >> 2 = 5
Left Shift (<<) Operators
The left shift(<<) is a binary operator that takes two numbers, left shifts the bits of the first operand, and the second operand decides the number of places to shift. In other words, left-shifting an integer “a” with an integer “b” denoted as ‘(a<<b)’ is equivalent to multiplying a with 2^b (2 raised to power b).
Syntax
a << b;
where,
a
is the integer value to be shifted.b
specifies how many positions to shift the bits.
Example: Let’s take a=21; which is 10101 in Binary Form. Now, if “a is left-shifted by 1” i.e a = a << 1 then a will become a = a * ( 2 ^ 1). Thus, a = 21 * (2 ^ 1) = 42 which can be written as 10100.
But if the size of the data type of a is only 5 bits, then the first bit will be discarded we will be left with a = 10, which is 01010 in binary. It is shown in the below image.
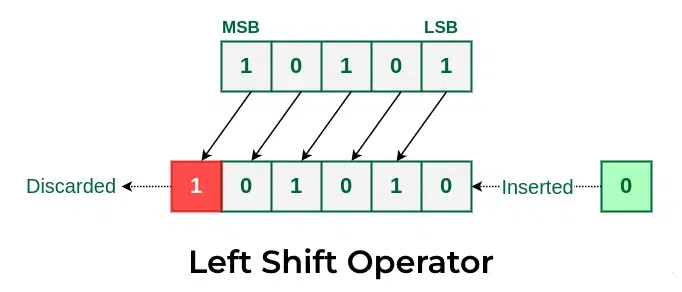
Example of Left Shift Operator
C++
// C++ Program to demonstrate use
// of left shift operator
#include <iostream>
using namespace std;
int main() {
// a = 21(00010101)
unsigned char a = 21;
// The result is 00101010
cout << "a << 1 = " << (a << 1);
return 0;
}
C
// C Program to demonstrate use
// of left shift operator
#include <stdio.h>
int main() {
// a = 21(000010101)
unsigned char a = 21;
// The result is 00101010
printf("a << 1 = %d\n", (a << 1));
return 0;
}
Applications of Left Shift Operator
- Multiplication by Powers of Two: Left shifting a number by
n
positions is equivalent to multiplying it by 2^n
and is much faster than normal multiplication - Efficient Calculations: Used in performance-critical applications where arithmetic operations need to be fast.
- Bit Manipulation: Common in low-level programming, such as embedded systems and hardware interfacing.
To gain a deeper understanding of bitwise operations and how they improve performance, our Complete C++ Course offers detailed tutorials on using operators efficiently in C++.
Right Shift(>>) Operators
Right Shift(>>) is a binary operator that takes two numbers, right shifts the bits of the first operand, and the second operand decides the number of places to shift. In other words, right-shifting an integer “a” with an integer “b” denoted as ‘(a>>b)‘ is equivalent to dividing a with 2^b.
Syntax
a >> b;
where,
a
is the integer value to be shifted.b
specifies how many positions to shift the bits.
Example: Let’s take a=21; which is 10101 in Binary Form. Now, if a is right shifted by 1 i.e a = a >> 1 then a will become a=a/(2^1). Thus, a = a/(2^1) = 10 which can be written as 1010.
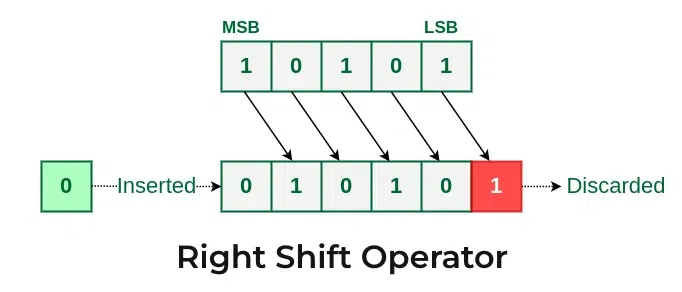
Example of Right Shift Operator
C++
// C++ Program to demonstrate
// use of right-shift operator
#include <iostream>
using namespace std;
int main() {
// a = 21(00010101)
unsigned char a = 21;
// The result is 00001010
cout << "a >> 1 = " << (a >> 1);
return 0;
}
C
// C Program to demonstrate
// use of right-shift operator
#include <stdio.h>
// Driver code
int main()
{
// a = 21(00010101)
unsigned char a = 21;
// The result is 00001010
printf("a >> 1 = %d\n", (a >> 1));
return 0;
}
Applications of Right Shift Operators
- Division by Powers of Two: Right shifting a number by
n
positions is equivalent to dividing it by 2^n
and it is very fast. - Efficient Calculations: Used in performance-critical applications for fast division operations.
- Bit Manipulation: Useful in extracting specific bits from data, common in data compression and cryptography.
Important Points of Shift Operators
1. The left-shift and right-shift operators should not be used for negative numbers. The result of is undefined behavior if any of the operands is a negative number. For example, results of both 1 >> -1 and 1 << -1 is undefined.
C++
// C++ program to show behaviour of shift operators for
// negative values
#include <iostream>
using namespace std;
int main()
{
// left shift for negative value
cout << "2 << -5 = " << (2 << -5) << endl;
// right shift for negative value
cout << "2 >> -5 = " << (2 >> -5) << endl;
return 0;
}
C
// C program to show behaviour of shift operators for
// negative values
#include <stdio.h>
int main()
{
// left shift for negative value
printf("2 << -5 = %d\n", (2 << -5));
// right shift for negative value
printf("2 >> -5 = %d", (2 >> -5));
return 0;
}
Output2 << -5 = 0
2 >> -5 = 64
2. If the number is shifted more than the size of the integer, the behavior is undefined. For example, 1 << 33 is undefined if integers are stored using 32 bits. For bit shift of larger values 1ULL<<62 ULL is used for Unsigned Long Long which is defined using 64 bits that can store large values.
C++
// c++ program to demonstrate the behaviour of bitwise
// shift operators for large values
#include <iostream>
using namespace std;
int main()
{
int N = 3;
// left shift by 65 digits
cout << "3 << 65" << (3 << 65) << endl;
return 0;
}
C
// c program to demonstrate the behaviour of bitwise
// shift operators for large values
#include <stdio.h>
int main()
{
int N = 3;
// left shift of 65 digits
printf("3 << 65 = %d", (3 << 65));
return 0;
}
3. The left-shift by 1 and right-shift by 1 are equivalent to the product of the first term and 2 to the power given element(1<<3 = 1*pow(2,3)) and division of the first term and second term raised to power 2 (1>>3 = 1/pow(2,3)) respectively.
C++
// C++ program to get the shifted values using pow()
#include <cmath>
#include <iostream>
using namespace std;
int main()
{
cout << "2^5 using pow() function" << pow(2, 5) << endl;
cout << "2^5 using leftshift" << (1 << 5) << endl;
return 0;
}
C
// C program for the above approach
#include <math.h>
#include <stdio.h>
int main()
{
printf("2^5 using pow() function: %.0f\n", pow(2, 5));
printf("2^5 using left shift: %d\n", (1 << 5));
return 0;
}
// This code is contributed Prince Kumar
Output2^5 using pow() function32
2^5 using leftshift32
Must Read: Bitwise Operators in C/C++