TypeScript Function Type Expressions
Last Updated :
31 Oct, 2023
In this article, we are going to learn about TypeScript Function Type Expressions in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, a function type expression represents the type of a function, including its parameter types and return type. Function type expressions can be used to define and annotate the types of functions, which helps in enforcing type safety and providing better code documentation. These types are syntactically similar to arrow functions.
Syntax:
(parameter1: type1, parameter2: type2, ...) => returnType
Where-
- (parameter1: type1, parameter2: type2, ...): This part defines the parameters that the function expects. Each parameter is followed by a colon: and its corresponding type. You can specify multiple parameters separated by commas.
- =>: The fat arrow => separates the parameter list from the return type.
- returnType: This specifies the type of the value that the function returns
Example 1: In this example, We define a function type expression called MathOperation using the arrow function-like syntax. It specifies that any function of this type should take two numbers (a and b) as parameters and return a number. We then create two functions, add and subtract, that conform to the MathOperation type. Both functions take two numbers and return the result of addition and subtraction, respectively.
JavaScript
// Define a function type expression
// using arrow function-like syntax
type MathOperation = (a: number, b: number) => number;
// Create a function that conforms
// to the MathOperation type
const add: MathOperation = (a, b) => a + b;
const subtract: MathOperation = (a, b) => a - b;
// Use the defined functions
console.log(add(5, 3));
console.log(subtract(10, 4));
Output:
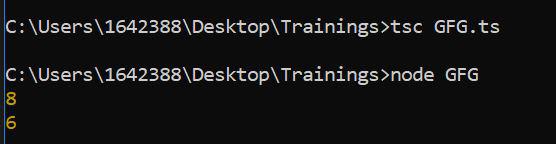
Example 2: In this example,We define a function type expression called StringManipulator using the arrow function-like syntax. It specifies that any function of this type should take a string (input) as a parameter and return a string.We create two functions, capitalize and reverse, that conform to the StringManipulator type. The capitalize function capitalizes the first letter of a string, and the reverse function reverses the characters in a string.
JavaScript
// Define a function type expression
// using arrow function-like syntax
type StringManipulator = (input: string) => string;
// Create a function that conforms
// to the StringManipulator type
const capitalize: StringManipulator = (input) => {
return input.charAt(0).toUpperCase() + input.slice(1);
};
const reverse: StringManipulator = (input) => {
return input.split('').reverse().join('');
};
// Use the defined functions
const originalString = 'GeeksforGeeks is a Computer Science Portal';
const capitalizedString = capitalize(originalString);
console.log(capitalizedString);
const reversedString = reverse(originalString);
console.log(reversedString);
Output:

Conclusion: In this article, we learnt that Function type expressions can be used to define and annotate the types of functions, which helps in enforcing type safety and providing better code documentation. These types are syntactically similar to arrow functions.